5 Programming Principles To Live By
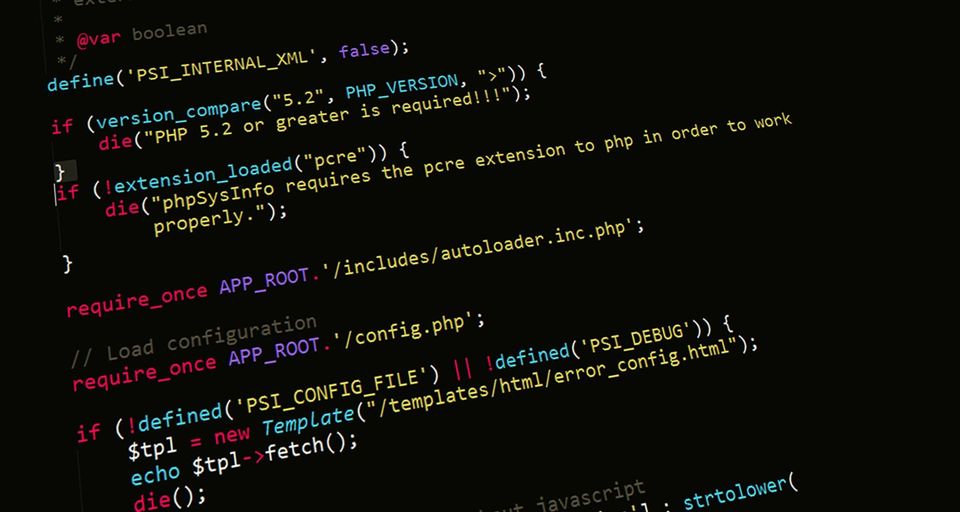
As programmers, we try to follow certain rules and patterns to govern how we write code that delivers software. While these patterns might not make the software 'work better', they are important for the maintenance and longevity of the application and contribute to easily newer developers can take up the baton and run.
Never forget that code should be reusable and maintainable. The following are 5 principles that will help you to maintain those standards.
1. KISS (Keep It 'Simple Stupid' or 'Super Simple')
This is first on the list because it can determine how easily another developer can come and see and understand your code. Frankly, it also affect how well you can understand it after a weekend off.
Commenting code is good, but when code is written in a simple and efficient manner, it can speak for itself.
Always ensure that your code is solving specific problems, using the simplest and most understandable techniques possible.
2. DRY (Don't Repeat Yourself)
Sometimes, it seems harmless to repeat a block of code. But then, we need the block another time...and in another place. Then the requirement changes, that's 5 places that this block of code was repeated and 5 additional bouts of maintenance work.
This is why methods and then classes exist. Try to abstract code that will be used multiple times and place in a central location that other places and developers can access it. This makes it easier to maintain in the long run and takes pressure off of maintenance and refactoring activities.
3. YAGNI (You Aren't Going To Need It)
I have seen too many times where the super star developer on the team writes some code for an edge case. Edge case meaning, maybe it will...maybe it won't. Sometimes, the code is no longer needed and they just comment it out and leave it there...just in case.
If you don't need code now, then don't write it. That is time that could be better spent ensuring that the current objectives are being met with relevant code. Also, don't develop attachment syndrome to code that is being deprecated. If you are using source control, then you can always retrieve the version of the code after you have removed (not just commented...but removed) it from the code base.
This ultimately leads to much cleaner code and readability.
4. Separation of Concern
The foundation of this principle is that you should always ensure that your blocks of code are dealing with one thing at a time. In practice, this isn't always easy to accomplish based on the framework or even language you are using, but it is something to always remember.
Here are simple examples of how this can be ensured:
- A function should complete one operation.
Don't have it doing 2 or 3. eg. Sum(int num1, int num2) - Anything written in that function should be towards a single outcome.
eg. Sum(4, 1) returns 5. Not 4 (Product) and .25 (quotient) - All functions related to common tasks, should be put into one class.
class Calculator
{
int Sum(int num1, int num2){...}
int Difference(int num1, int num2){...}
int Product(int num1, int num2){...}
int Quotient(int num1, int num2){...}
} - All related classes get compiled into *one library *
I am sure you get the point, ensure that your code has one major task to completed per block.
5. Do A Design Document
Sometimes in our zeal, we rush head first into development based on a vision and then end up making major changes in the middle of it all.
It is important to have a clear design and I would extend that and say, design document. This helps you to think ahead and realize potential gaps beforehand and have a clear goal and path in mind.
Conclusion
Sometimes it is not about being a great programmer, it is more about being proficient, but adhering to good habits.
You might not always get it right the first time, but always be willing to critique yourself and accept feedback as you grow in your profession.
Member discussion